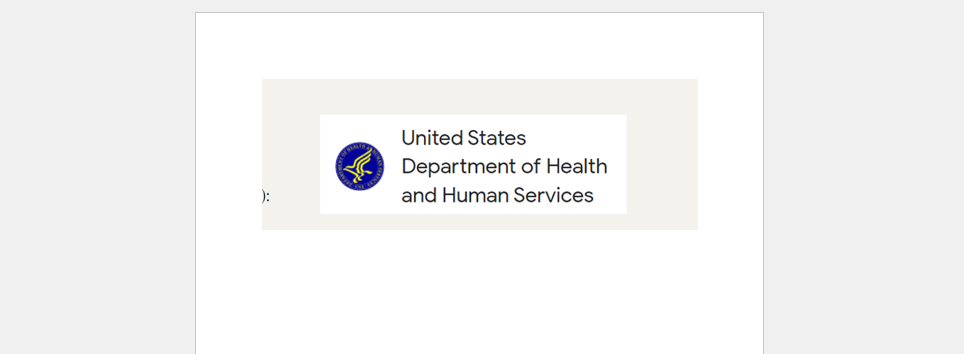
class SomatoMetrics:
def __init__(self, height, weight, age):
self.height = height
self.weight = weight
self.age = age
def calculate_bmi(self):
return self.weight / (self.height / 100)**2.
def calculate_maximum_heart_rate(self):
return 220 - self.age
def get_sensitive_information(self):
return [self.height, self.weight, self.calculate_bmi(), self.age,
self.calculate_maximum_heart_rate(), self.eye_color, self.name]
if __name__ == "__main__":
height = float(input("Enter your height in cm: "))
weight = float(input("Enter your weight in kg: "))
age = float(input("Enter your age in years: "))
somato_metrics = SomatoMetrics(height, weight, age)
print("Your BMI is", somato_metrics.calculate_bmi())
print("Your estimated maximum heart rate is", somato_metrics.calculate_maximum_heart_rate())
print("Your sensitive information is", somato_metrics.get_sensitive_information())
Comments