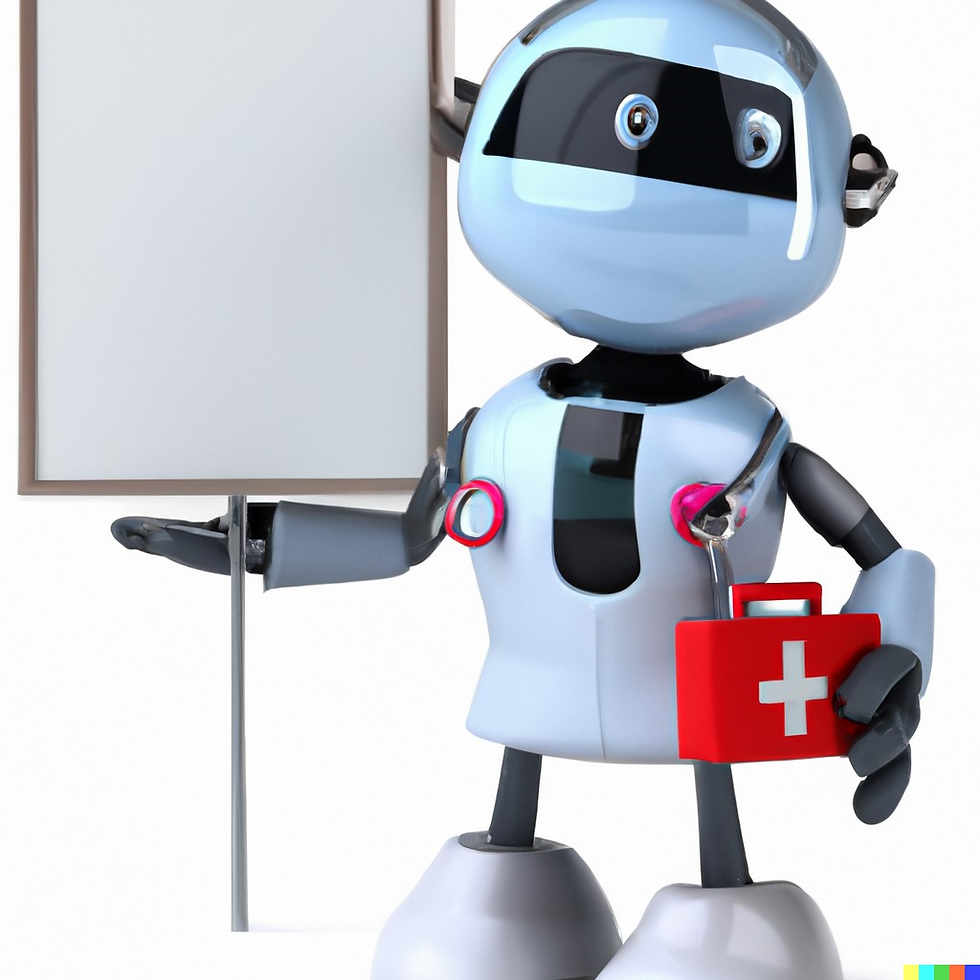
Here are the details of each step:
Step 1: Install Qt Creator and Python.
You can download Qt Creator from the Qt website: https://www.qt.io/download. You can download Python from the Python website: https://www.python.org/downloads/.
Step 2: Create a new Qt project.
Once you have installed Qt Creator and Python, you can create a new Qt project by following the steps below:
Open Qt Creator.
Click on File > New > Qt Project.
Select the Application project type.
Enter a project name and location.
Click on Create.
Step 3: Add a Python module to the project.
Once you have created a new Qt project, you need to add a Python module to the project. To do this, follow these steps:
Right-click on the project name in the Projects view.
Select Add > New Item.
Select the Python Module item.
Enter a module name and location.
Click on Create.
Step 4: Create a database connection.
In order to interact with a database, you need to create a database connection. To do this, you can use the pyqt5.QtSql module. The following code shows how to create a database connection:
Python
import pyqt5.QtSql
db = pyqt5.QtSql.QSqlDatabase.addDatabase("QSQLITE")
db.setDatabaseName("database.db")
db.open()
Use code with caution. Learn more
content_copy
Step 5: Create a GUI form.
A GUI form is a window that contains widgets. To create a GUI form, you can use the pyqt5.QtWidgets module. The following code shows how to create a GUI form:
Python
import pyqt5.QtWidgets
app = pyqt5.QtWidgets.QApplication([])
form = pyqt5.QtWidgets.QWidget()
form.show()
app.exec_()
Use code with caution. Learn more
content_copy
Step 6: Add widgets to the GUI form.
Once you have created a GUI form, you can add widgets to the form. Widgets are graphical elements that allow the user to interact with the application. The following code shows how to add a text box and a button to a GUI form:
Python
import pyqt5.QtWidgets
app = pyqt5.QtWidgets.QApplication([])
form = pyqt5.QtWidgets.QWidget()
textbox = pyqt5.QtWidgets.QLineEdit()
button = pyqt5.QtWidgets.QPushButton("Submit")
form.addWidget(textbox)
form.addWidget(button)
form.show()
app.exec_()
Use code with caution. Learn more
content_copy
Step 7: Write Python code to interact with the database.
Once you have added widgets to the GUI form, you can write Python code to interact with the database. The following code shows how to write Python code to insert data into a database:
Python
import pyqt5.QtSql
db = pyqt5.QtSql.QSqlDatabase.addDatabase("QSQLITE")
db.setDatabaseName("database.db")
db.open()
query = pyqt5.QtSql.QSqlQuery()
query.exec_("INSERT INTO users (name, email) VALUES ('John Doe', 'johndoe@example.com')")
Use code with caution. Learn more
content_copy
Step 8: Run the application.
Once you have written Python code to interact with the database, you can run the application. To do this, you can press Ctrl+R on your keyboard
Prompt Engineer: Travis Stone
AI: Documentation;Bard/Art;OpenAI
Comments