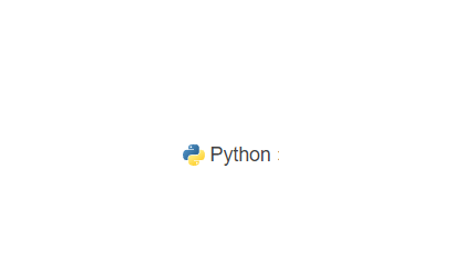
Functional Programming in Python: Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. In Python, functional programming can be achieved through the use of higher-order functions, lambda expressions, and immutable data structures.
1. for Loop:
Sequence: A collection of items, such as a list, tuple, string, or dictionary, that you want to loop through.
Item: Each individual element within the sequence that the for loop processes one by one.
Iteration: The process of going through each item in the sequence one at a time during the loop execution.
Index: The position of an item in the sequence. In Python, indexing starts from 0.
Iterable: An object that can be iterated over, allowing the for loop to access its elements.
in Keyword: A keyword used in the for loop to specify the iterable being looped through.
Block of Code: The set of instructions indented under the for statement that will be executed for each item in the sequence.
for Loop Examples:
for Loop: The for loop in Python is used to iterate over a sequence (like a list, tuple, string, or dictionary) and perform a set of instructions for each item in the sequence. It's like going on a bike ride where you have a list of destinations to visit, and you ride your bike to each destination one by one.
Analogies:
Imagine you have a list of places to visit, like parks, cafes, and libraries. With a for loop, you can ride your bike to each place in the list and enjoy each location.
If you have a string containing the names of your friends, you can use a for loop to greet each friend individually while riding your bike down the street.
Syntax:
pythonCopy code
places_to_visit = ["Park", "Cafe", "Library"]
for place in places_to_visit:
# Ride the bike to the current placeprint("Visiting", place)
Example 2: Print Numbers from 1 to 5
pythonCopy code
for num in range(1, 6):
print(num)
Output:
Copy code
1
2
3
4
5
Example 3: Sum of Elements in a List
pythonCopy code
numbers = [10, 20, 30, 40, 50]
sum = 0for num in numbers:
sum += num
print("Sum:", sum)
Output:
makefileCopy code
Sum: 150
Example 4: Loop through a String
pythonCopy code
message = "Hello, Python!"for char in message:
print(char)
Output:
cssCopy code
H
e
l
l
o
,
P
y
t
h
o
n
!
2. while Loop:
Condition: A Boolean expression that determines whether the loop should continue running or stop.
Loop Continuation: The loop keeps running as long as the condition evaluates to True.
Loop Termination: The loop stops running when the condition evaluates to False.
Pre-Test Loop: The condition is checked at the beginning of the loop iteration.
Post-Test Loop: The condition is checked at the end of the loop iteration, ensuring the loop executes at least once.
Infinite Loop: A loop that never terminates because its condition is always True.
Break Statement: A control statement used inside the loop to immediately exit the loop when a specific condition is met.
While Loop Examples:
while Loop: The while loop in Python is used to repeatedly execute a set of instructions as long as a specific condition is true. It's like riding a bike in circles around a park until it gets dark or you decide to stop.
Analogies:
Imagine you want to ride your bike in the park until you feel tired. You would continue riding in circles until you're ready to stop. The while loop does something similar by repeating a block of code until a condition is no longer true.
If you want to keep riding your bike to collect coins until you have a certain amount, you would use a while loop that continues collecting coins until the desired amount is reached.
Syntax:
pythonCopy code
coins_collected = 0
desired_coins = 10while coins_collected < desired_coins:
# Ride the bike and collect a coin
coins_collected += 1print("Collected a coin. Total coins:", coins_collected)
Example 2: Countdown Timer
pythonCopy code
countdown = 5while countdown > 0:
print(countdown)
countdown -= 1print("Blastoff!")
Output:
Copy code
5
4
3
2
1
Blastoff!
Example 3: User Input Validation
pythonCopy code
password = "secret"
input_password = ""while input_password != password:
input_password = input("Enter the password: ")
print("Access granted!")
Output:
mathematicaCopy code
Enter the password: abcEnter the password: 123Enter the password: secretAccess granted!
3. do-while Loop (Simulated in Python using while):
Loop Body: The set of instructions inside the loop that will be executed at least once.
Condition: The Boolean expression checked at the end of each iteration to determine if the loop should continue or terminate.
Iteration: The process of executing the loop body and checking the condition afterward.
Simulated do-while Loop: In Python, this loop is achieved by using a while loop with a condition that is always True, and using a break statement to exit the loop when the desired condition is met.Python is a high-level, interpreted, and dynamically-typed programming language known for its simplicity and readability. It supports both functional and object-oriented programming paradigms, providing developers with flexibility in designing their applications.
do-while Loop (Simulated in Python using while) Examples:
do-while Loop (Simulated in Python using while): Python doesn't have a traditional do-while loop like some other programming languages, but you can simulate it using a while loop with a condition at the end. The code inside the loop will always execute at least once before checking the condition, similar to how you ride your bike and then check if you want to continue.
Analogies:
Suppose you want to ride your bike at least once around the block before deciding whether to continue or not. You would ride once, and then if you still have energy, you'd continue riding. This is similar to a do-while loop.
Syntax:
pythonCopy code
# Simulate a do-while loop
energy = 5while True:
# Ride the bike at least onceprint("Riding the bike...")
energy -= 1# Check if you want to continueif energy <= 0:
breakEnsure Positive Number Input
pythonCopy code
number = 0while True:
number = int(input("Enter a positive number: "))
if number > 0:
breakelse:
print("Invalid input. Try again.")
print("You entered:", number)
Output:
lessCopy code
Enter a positive number: 0Invalid input. Try again.
Enter a positive number: -5Invalid input. Try again.
Enter a positive number: 10You entered: 10
Example 2: Guess the Number Game
pythonCopy code
import random
secret_number = random.randint(1, 100)
guess = 0while True:
guess = int(input("Guess the secret number (1-100): "))
if guess == secret_number:
print("Congratulations! You guessed the secret number.")
breakelif guess < secret_number:
print("Try again. The secret number is higher.")
else:
print("Try again. The secret number is lower.")
Prompt Engineer: Travis Stone
AI: OpenAI
Comments